Exercise 2: Close Dialog and Zoom
For this exercise let's iterate on improving the search functionality to:
- Close the search dialog by dispatching the
CloseDialogs
action when the backdrop is tapped/clicked. - Close the search dialog by dispatching the
CloseDialogs
action when a resort is selected. - Hide the sidenav by dispatching the
HideSidenav
action when a resort is selected. - Set the zoom level higher after a resort is selected.
To begin, checkout the 06-effects-exercise-1 branch:
git checkout 06-effects-exercise-2
🤫 The solution branch is available if you get stuck.
You can checkout the solution via:
git checkout 06-effects-exercise-2-solution
Goals
- Practice declaring actions.
- Practice dispatching actions from an effect.
Step 1: Close Dialog
For the first iteration we want to dispatch the CloseDialogs
action when the backdrop is tapped/clicked using an effect:
- Remove the
{ dispatch: false }
option in the@Effect
decorator for theopen
effect in src/app/state/dialog/dialog.effects.ts. - Set the
disableClose
configuration option for the dialog in src/app/state/dialog/dialog.effects.ts. This will disable the default behavior of closing the dialog when the backdrop is tapped/clicked. - Use the
switchMap()
operator to switch to the projected observable returned fromMatDialogRef.backdropClick()
. - Use the
map()
operator to return theCloseDialogs
action.
For the next iteration we want to dispatch the CloseDialogs
action when the SelectResort
resort action is dispatched using an effect:
- Add a new
closeDialogOnSelect
property to theResortEffects
class and decorate it using the@Effect
decorator in src/app/state/resort/resort.effects.ts. - Use the
ofType()
operator to filter for theSeletResort
action. - Use the
map()
operator to return theCloseDialogs
action.
Step 2: Hide Sidenav
Dispatch the HideSidenav
action when the SelectResort
action is dispatched using an effect:
- Add a new
hideSidenavOnSelect
property to theResortEffects
class and decorate it using the@Effect
decorator in src/app/state/resort/resort.effects.ts. - Use the
ofType()
operator to filter for theSelectResort
action. - Use the
map()
operator to return theHidesidenav
action.
Step 3: Set Zoom
Dispatch the SetMapZoom
action when the SelectResort
action is dispatched using an effect:
- Add a new
setMapZoom
property to theResortEffects
class and decorate it using the@Effect
decorator in src/app/state/resort/resort.effects.ts. - Use the
ofType()
operator to filter for theSelectResort
action. - Use the
map()
operator to return theSetMapZoom
action.
Try it Out
Use the Redex Devtools to view the [Dialog] Close Dialog action being dispatched:
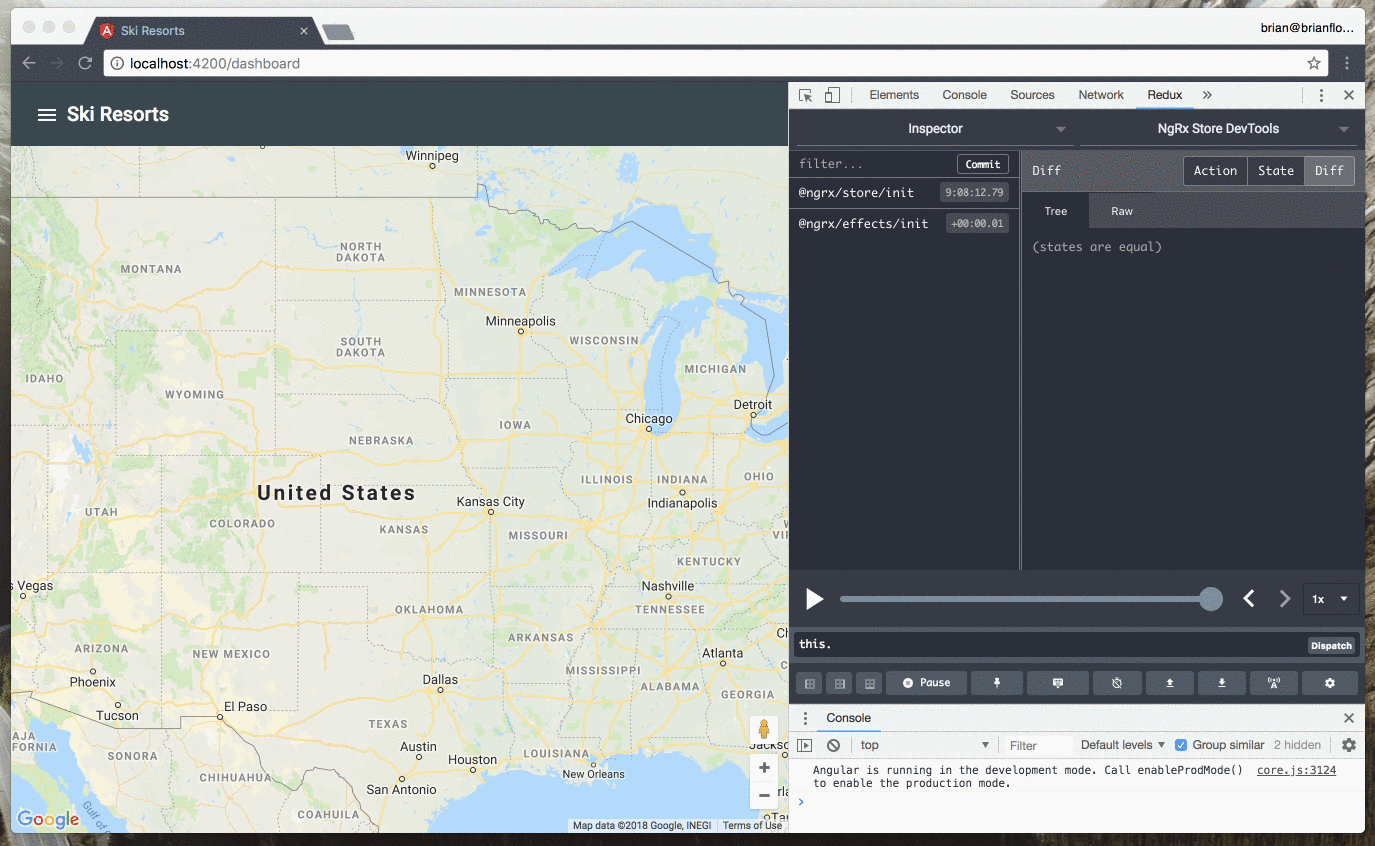
The [Sidenav] Hide Sidenav and [Map] Set Zoom actions are dispatched when a resort is selected:
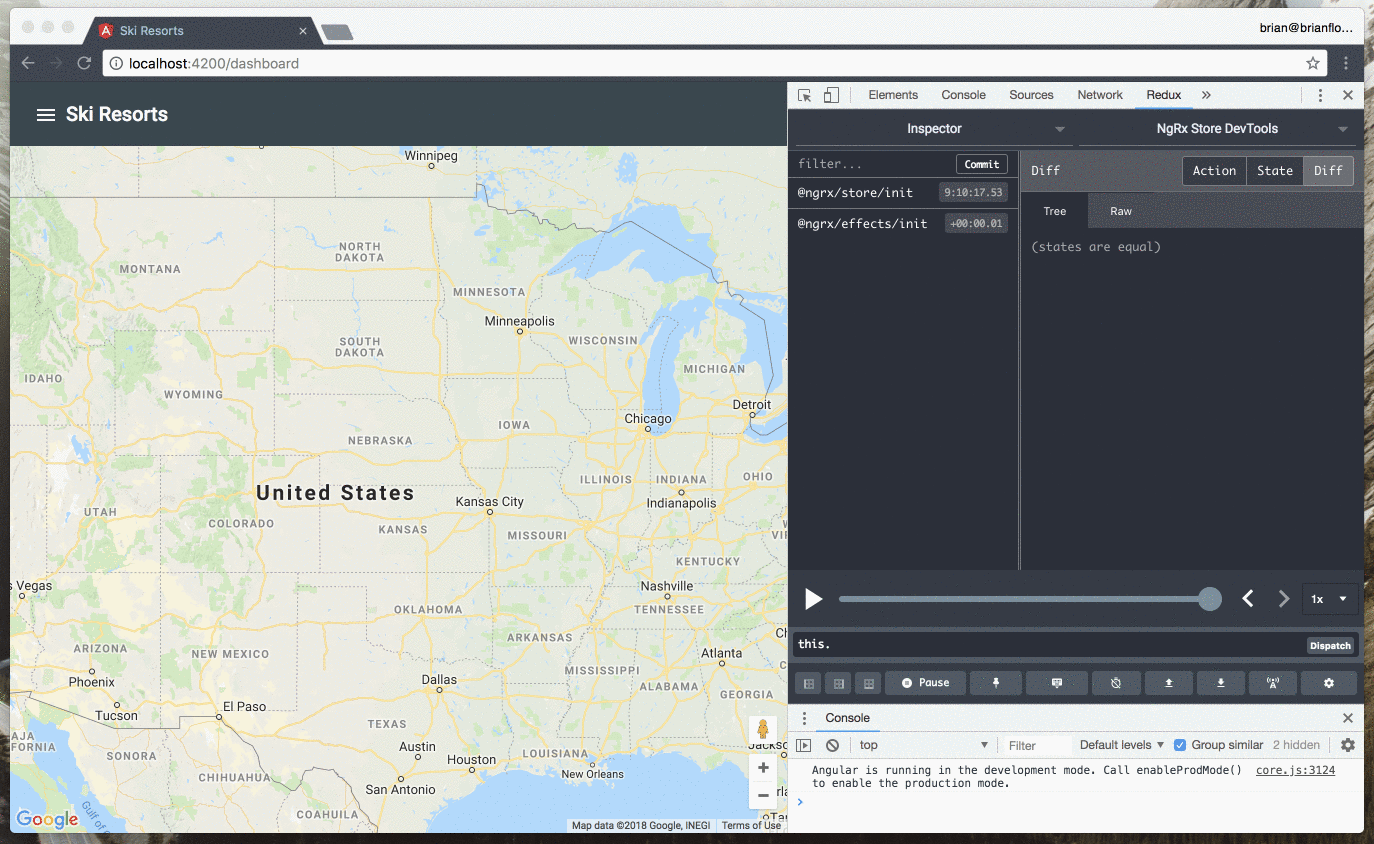